Install Go
Before we can begin writing Go code we’ll need to, would you believe it? Install Go
Install Text Editor
If you already have a text editor of choice, then you can skip this step. If you don’t know what a text editor is, then let me explain.
Software Engineers are modern day wizards.
How so?
- We can make updates anywhere in the world ๐ to a piece of software and everywhere in the world ๐ ๐ is affected.
- We can bend reality to our will by making software that can change physics, images ๐ , voices ๐น๐ฌ, appearances ๐ -> ๐
- We can summon, literally, tens of thousands of computers ๐ค๐ค๐ค๐ค๐ค๐ค to serve us in a moment’s notice.
OK, so programmers are wizards๐งโโ๏ธ ๐ง, what’s the point? Well, like anyone who practices the magic arts๐ง๐ง, you need a tool. Instead of using a wand ๐ช developers use text editors or IDEs (Integrated Development Environment). Text editors allow us to use our powers.
Time to choose your Text Editor
1. VSCode
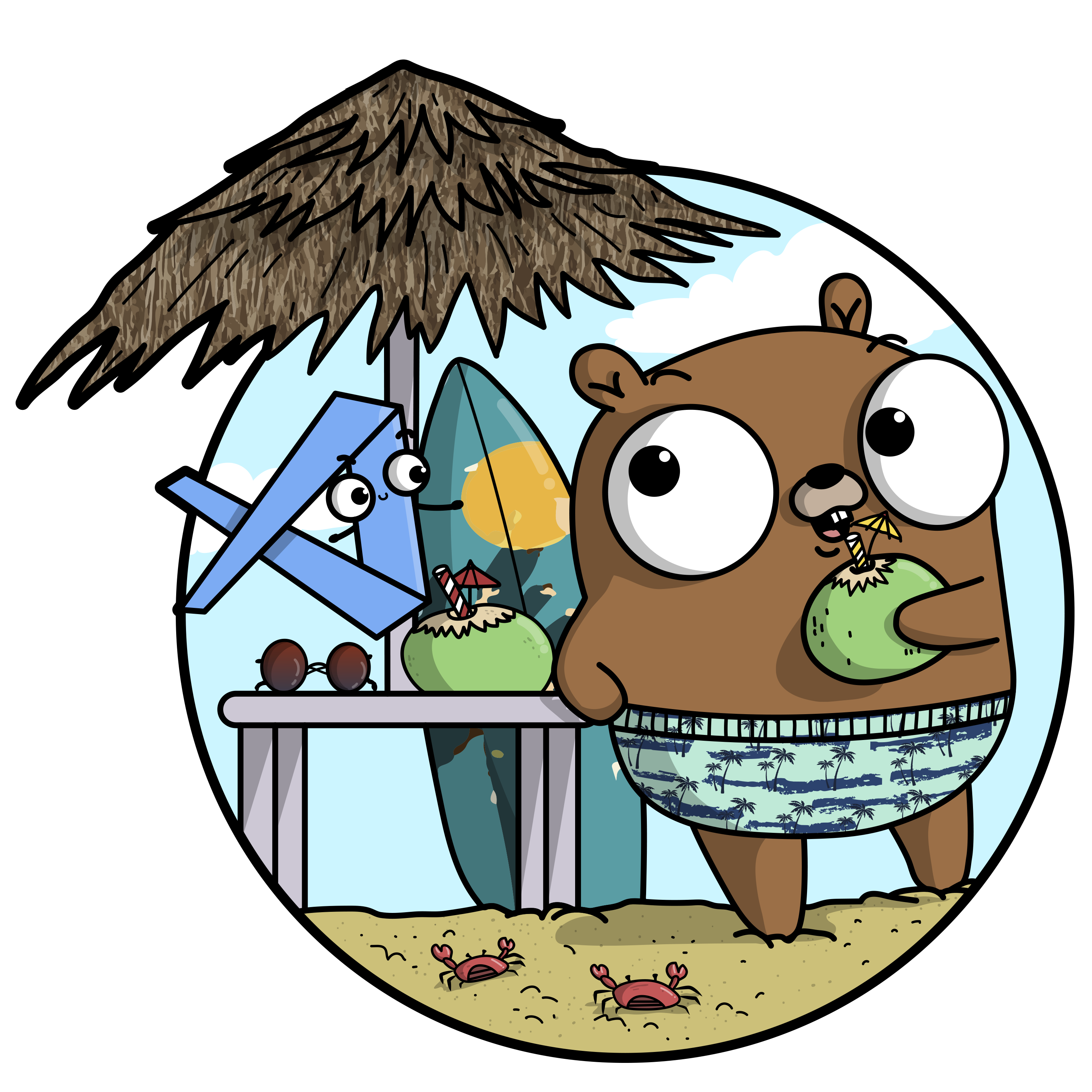
2. Vim
Vim Go by Egon Elbre- License
I use Neovim but if you’re a beginner, I highly recommend VSCode. Vim is a very, very powerful ๐ฆธโโ๏ธ text editor, but it is not beginner friendly. If you know what you’re doing and want to improve your speed and understanding as a developer give Neovim or Vim a try; Here’s an article to get started with Go in Vim or with VSCode you want to search for the Go extension and install it.
Setup
We have Go installed and we have our text editor, now it’s time to start
our first projectโ Are you excitedโ๏ธ I am ๐ From this point on I’ll assume
we’re beginners and using VSCode. Open VSCode and hit Ctrl+` (That’s
the backtick or the key right next to 1
on a QWERTY keyboard) to open the
Terminal
and
type the following in the terminal:
mkdir basics
– make a directory and name it basics (Our project name)cd basics
– change directory into our project basicsgo mod init basics
– initialize (start) a go module named basicsmkdir cmd
– make a directory and name it cmd (Idiomatic to do in Go)touch cmd/main.go
– make a file named main.go in the cmd directorycode -r .
– make VSCode update the working directory to our project
Now you should see a little directory named cmd
on the left. Open it
and click main.go
First Program
Now time to write your first Go
code.
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
Lets explain each line.
package main
Go
needs you to tell it where it should pack all of the functionality you’re
going to make in this file. You can have something like package hello
at the
top and have many files that have package hello
at the top of their files as
well. When Go
builds it that entire package gets… Well, packaged together
๐ Make sense? In other words grouped or bundled together. Think of noodles ๐
being put into a take out box ๐ฅก The files we make are the noodles ๐ and the
package
we declare is the take out box ๐ฅก
package main
is special because this is where Go
will begin execution of
your program. If you’re trying to run a Go
app you must have a package main
with a func main()
.
import "fmt"
fmt
is a package ๐ฆ! Just like the package main
we made one line above, but
it comes from the standard library ๐. The standard library is a place where
lots of amazing packages exists, all we have to do is import
them! Later
we’ll make our own packages and we can run them here in cmd/main
.
func main()
func main()
is the place where it all begins and it all ends ๐, your
application that is. Here is where you will start and end anything and
everything you want your program to do. So always remember if you want to go run
your application, it needs to be in package main
and it must be inside
of the func main()
function.
fmt.Println( ... )
Here we call the fmt
package that we imported to use it’s Println
function. Println
is short for print ๐จ๏ธline. It’s called that because it will
print a line of text to your terminal, also known as standard out. You can pass
lots of things to Println
, but we’ll see more of that in later lessons.
Run your very first program
Save the file and type go run cmd/main.go
into your terminal.
Playground
link
๐ฅณ๐ฅณ Congratulations! ๐๐๐ You’re officially a Gopher!
Why not celebrate and Make Your Own Gopher and if you want there’s a very supportive community over on Slack and Reddit and finally Golang Weekly ๐ฐ is great for getting ideas about what’s going on in the Go community.
Problems?
It’s very important that you’re in the basics
directory when you run go run cmd/main.go
. If you get an error message like: stat main.go: no such file or directory
that means you tried to execute something that wasn’t there! go
is
telling you,
I looked for the file
main.go
where you asked me to look and it’s not there! So it looks like I can’t run it.
So make sure you’re in the the basics
directory, you can find out by
typing pwd
in your terminal and pressing Enter/Return
If you’re still facing problems send an email to info@gophergo.dev